Generating a KML with data from postgis and libkml
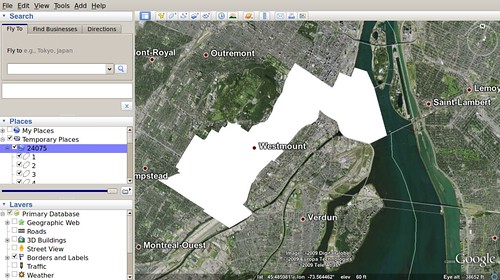
So right now I am working on an experimental project to produce a mashup with the electoral data down to the polling station level.
Right now, I imported the data from the Shapefile provided free of charge on Geogratis to a PostgreSQL w/ Postgis database.
I am then using the libkml library (with SWIG bindings) to merge the data from postgis. Postgis conveniently has a SQL function called "ST_AsKML()" that outputs the geometry as the corresponding KML code. Here is the code that I used:
#!/usr/bin/env python import sys import pg import kmlbase import kmldom import kmlengine def usage(): return 'usage: riding.py [fed_num]' def main(): if len(sys.argv) > 1: if len(sys.argv[1]) != 5: print usage() return False factory = kmldom.KmlFactory_GetFactory() docu = factory.CreateDocument() docu.set_name(sys.argv[1]) kml = factory.CreateKml() kml.set_feature(docu) conn = pg.connect('postgis', '127.0.0.1', 5432, None, None, 'DB_NAME', 'DB_PASS') rows = conn.query('SELECT pd_id, pd_num, pd_nbr_sfx, pd_type, adv_poll, ed_id, fed_num, ST_AsKML(the_geom) as boundary FROM pd308_a WHERE fed_num = %05d ORDER BY pd_num ' % int(sys.argv[1])) res = rows.dictresult() for poll in res: pl = factory.CreatePlacemark() pl.set_name(str(poll['pd_num'])) kmlfile,errors = kmlengine.KmlFile.CreateFromParse(poll['boundary']) mg = kmldom.AsMultiGeometry(kmlfile.get_root()) pl.set_geometry(mg) docu.add_feature(pl) print kmldom.SerializePretty(kml) else: print usage() if __name__ == '__main__': main()
Run this on the command line with python (my version is 2.6). This code generates a KML file with all the different polls as separate placemarks for any given federal riding. For now, there is no styling or balloons.
Edit (2009-08-17): Here is the result if I randomize the polygon's colors.
Download this generated KML (for Westmount--Ville-Marie - 24075)
Leave a comment